newind2000
2015. 6. 16. 17:38
================================ Outline ====================================
다중상속
----------------------------------------------------------------------------
다중 상속을 받을 시 클래스 내부 변수가 겹칠 때는 스코프(::)를 사용하여 변수가 속한 위치를 명기해 주어야 한다.
다중 상속 시 멤버를 중복해지 상속하지 않고 한 번만 상속하기 위해서는 virtual 키워드를 사용하면 된다.
교재 p/286 클래스 - 포함 예제,
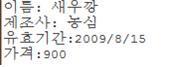
#include <iostream> #include <stdio.h> #include <string.h>
using namespace std;
class Date { protected: int year, month, day; public: Date(int y, int m, int d) { year = y; month = m; day = d; } void OutDate() { printf("%d/%d/%d", year, month, day); } };
class Product { private: char Name[64]; char Company[32]; Date ValidTo; int Price;
public: Product(const char *aN, const char *aC, int y, int m, int d, int aP) : ValidTo(y, m, d) { strcpy(Name, aN); strcpy(Company, aC); Price = aP; }
void OutProduct() { printf("이름: %s \n", Name); printf("제조사: %s \n", Company); printf("유효기간:"); ValidTo.OutDate(); puts(""); printf("가격:%d\n", Price); } };
int main(void) { Product S("새우깡", "농심", 2009,8,15,900); S.OutProduct(); return 0; }
|
교재 p/289 private 상속 예제,
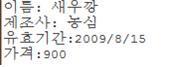
#include <iostream> #include <stdio.h> #include <string.h>
using namespace std;
class Date { protected: int year, month, day; public: Date(int y, int m, int d) { year = y; month = m; day = d; } void OutDate() { printf("%d/%d/%d", year, month, day); } };
class Product : private Date { private: char Name[64]; char Company[32]; int Price;
public: Product(const char *aN, const char *aC, int y, int m, int d, int aP) : Date(y, m, d) { strcpy(Name, aN); strcpy(Company, aC); Price = aP; } void OutProduct() { printf("이름: %s \n", Name); printf("제조사: %s \n", Company); printf("유효기간:"); OutDate(); puts(""); printf("가격:%d\n", Price); } };
int main(void) { Product S("새우깡", "농심", 2009,8,15,900); S.OutProduct(); return 0; }
|